Wednesday, January 28, 2015
Android beginner tutorial Part 44 ProgressDialog with spinner
A ProgressDialog is a Dialog that indicates a process - loading, downloading, etc. There are two ways of ProgressDialog appearances - one displays a spinner, the other one displays a progress bar. Today well learn about the first one.
Firstly we add a Button to our activity_main.xml layout:
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
tools:context=".MainActivity" >
<Button
android:id="@+id/testButton"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:text="Call an ProgressDialog"
/>
</LinearLayout>
In MainActivity.java class, the first thing we need to do is disable screen rotation. When the screen orientation changes, the Activity gets redrawn completely. All Dialogs that are open get closed too. However, all the Threads that are running dont stop. We are going to create a Thread when the button is clicked, which runs for 3 seconds and then closes the ProgressDialog. So, if the device is rotated and the screen changes orientation, the ProgressDialog gets closed but the Thread keeps running. When it stops, it will crash the application because it will try to close a ProgressDialog that doesnt exist.
There are a few way to prevent this, in this case I do it by just preventing screen rotation.
setRequestedOrientation(ActivityInfo.SCREEN_ORIENTATION_PORTRAIT);
Then I add a click event listener to display the dialog and create a Thread that sleeps for 3000 milliseconds and closes the dialog. Create the dialog using ProgressDialog.show() method, provide it with context and 2 text messages. You can close the dialog using dismiss() method. Dont forget to start() the Thread you create:
Button button = (Button)findViewById(R.id.testButton);
button.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
final ProgressDialog myDialog = ProgressDialog.show(MainActivity.this, "Loading...", "Please wait!");
new Thread(new Runnable(){
@Override
public void run(){
try{
Thread.sleep(3000);
}catch(Exception e){}
myDialog.dismiss();
}
}).start();
}
});
Full code:
package com.kircode.codeforfood_test;
import android.app.Activity;
import android.app.ProgressDialog;
import android.content.pm.ActivityInfo;
import android.os.Bundle;
import android.view.Menu;
import android.view.View;
import android.widget.Button;
public class MainActivity extends Activity{
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setRequestedOrientation(ActivityInfo.SCREEN_ORIENTATION_PORTRAIT);
setContentView(R.layout.activity_main);
Button button = (Button)findViewById(R.id.testButton);
button.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
final ProgressDialog myDialog = ProgressDialog.show(MainActivity.this, "Loading...", "Please wait!");
new Thread(new Runnable(){
@Override
public void run(){
try{
Thread.sleep(3000);
}catch(Exception e){}
myDialog.dismiss();
}
}).start();
}
});
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
// Inflate the menu; this adds items to the action bar if it is present.
getMenuInflater().inflate(R.menu.activity_main, menu);
return true;
}
}
Results:
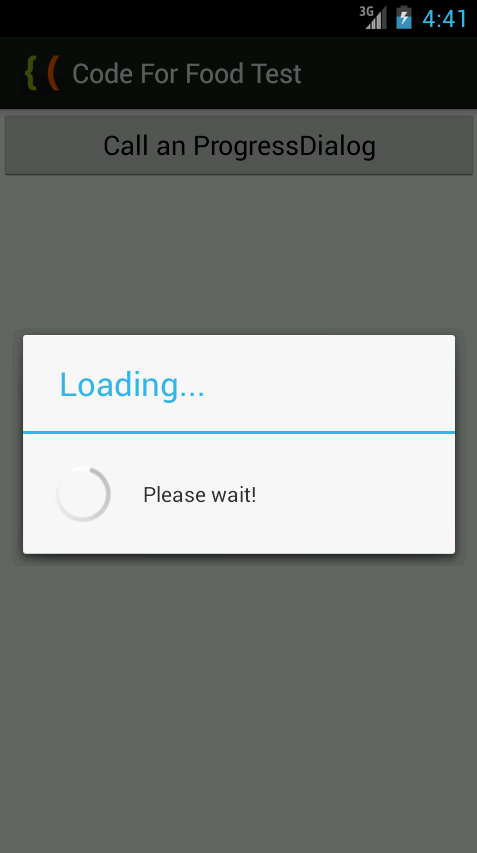
Thanks for reading!
Subscribe to:
Post Comments (Atom)
No comments:
Post a Comment
Note: Only a member of this blog may post a comment.