Friday, January 30, 2015
Creating a Pentomino game using AS3 Part 23
The Saved Levels screen is basically a browser for levels that are stored in the users local Shared Object.
Go to the main menu screen and add a new button with an id "btn_saved". Go to main_menu.as script file and add a click event handler for this button, which directs the user to the fourth frame:
package
{
import flash.display.MovieClip;
import flash.events.MouseEvent;
/**
* Open-source pentomino game engine
* @author Kirill Poletaev
*/
public class main_menu extends MovieClip
{
public function main_menu()
{
(root as MovieClip).stop();
btn_play.addEventListener(MouseEvent.CLICK, doPlay);
btn_editor.addEventListener(MouseEvent.CLICK, doEditor);
btn_saved.addEventListener(MouseEvent.CLICK, doSaved);
}
private function doPlay(evt:MouseEvent):void {
(root as MovieClip).gotoAndStop(2);
}
private function doEditor(evt:MouseEvent):void {
(root as MovieClip).gotoAndStop(3);
}
private function doSaved(evt:MouseEvent):void {
(root as MovieClip).gotoAndStop(4);
}
}
}
Go to the 4th frame on the main timeline in Flash and create a new MovieClip there. Give the new MC a class path saved_levels.
The saved levels screen consists of 3 parts - header, body and footer:
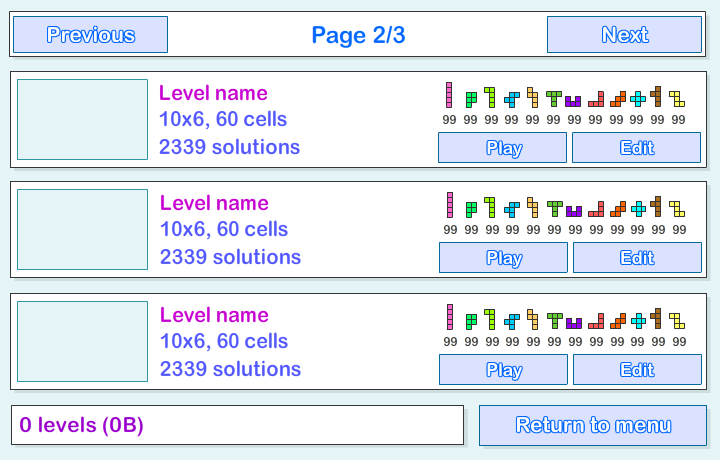
The header contains info about current page of the level browser and buttons to navigate through the pages.
The body of the screen contains up to 3 level items. Each level item is a saved_item object instance which I will talk about in details shortly.
The footer contains info about how many levels in total there are, how much space on local drive they all take and a button to return to main menu.
You need to add at least 5 objects right now - btn_previous and btn_next buttons in the header, tPage dynamic text field in the header, tInfo dynamic text field in the footer and a btn_back button in the footer.
As I said before, each item level is an instance of a saved_item object. Create a new MovieClip with class path saved_item and add the following objects: a rectangular MovieClip with id levelPreview; 3 dynamic text fields: tTitle, tSubtitle, tSubtitle2; 12 dynamic text fields with ids tShape1, tShape2, ... , tShape12; buttons btn_play and btn_edit.
The 3 dynamic text fields will be used to display level name, its statistics and number of solutions. The levelPreview object will be used to display the level grid. The 12 tShape text fields will be used to display how many of each shape is available. The two buttons do exactly what the name suggests - play the level and edit the level.
Add 3 of saved_item object instances to the screen.
Now create a new class saved_levels.as.
Right now we will just add functionality to the Back button and display the SharedObject data. Were storing an array called "levels" in a SharedObject and read its length to see how many levels there are. If the array is null, then there are 0 levels. We can use the size property to see how many bytes the object currently takes.
package
{
import flash.display.MovieClip;
import flash.events.MouseEvent;
import flash.net.SharedObject;
/**
* Open-source pentomino game engine
* @author Kirill Poletaev
*/
public class saved_levels extends MovieClip
{
private var savedLevels:SharedObject;
public function saved_levels()
{
savedLevels = SharedObject.getLocal("myLevels");
btn_back.addEventListener(MouseEvent.CLICK, doBack);
tInfo.text = ((savedLevels.data.levels == null)?("0"):(savedLevels.data.levels.length)) + " levels (" + savedLevels.size + "B)";
}
private function doBack(evt:MouseEvent):void {
(root as MovieClip).gotoAndStop(1);
}
}
}
Thats all for today.
Thanks for reading! The results so far:
Subscribe to:
Post Comments (Atom)
No comments:
Post a Comment
Note: Only a member of this blog may post a comment.